Tree¶
The tree control can be introduced as a control to the whole page in the Aurena client, which enables the data driven navigation.
Figure 1 - A Sample Tree
Variations¶
None.
When to use¶
The tree control can be used when data driven navigation is needed. That is, when different pages need to be loaded depending on the selected record, then the tree control can be used to achieve it.
How to use¶
The tree control consists of a main branch and sub branches, where each branch can have one or more nodes.
The main branch of the tree is called the main record selector, which is the same as the record selector in an ordinary Aurena page.
The main record selector consists of all the main nodes of the tree, the root nodes. Each root node in the main branch can be expanded in to several other sub branches, and each branch has child nodes in it. The last branch consists of leaf nodes, which cannot be expanded further.
When a record is selected from the main record selector an expand icon (refer to Figure 1) appears, and when clicking this icon it expands each root node to a tree. When clicking further on each node, the user can traverse through the branches up to the leaf nodes (refer to Figure 2).
The content area contains the data loaded for the selected record of the main record selector. When a user selects records through the nodes, the data gets loaded and is displayed accordingly in the content area.
Figure 2 - Tree structure
The following code describes the structure of a basic tree control.
tree <name> using <entityset> {
label = "<label>";
selector <selector ref>; //Main selector ref.
//Root node definition
rootnode {
label = "<node label>"; //Display text of the node (The value needs to be an interpolating string)
//Where to navigate when user clicks on the one
navigate {
page {
//Filter is mandatory for this navigation
filter(parameter, parameter);
}
}
//Possible child nodes of current node
connections {
node <root node name>(<Array ref or datasource for root node>)
node <node 1 name>(<Array ref or datasource for node 1>);
node <node 2 name>(<Array ref or datasource for node 2>);
....
node <node n name>(<Array ref or datasource for node n>);
}
}
//Node 1 definition
node <node 1 name> for <entity>{
label = "<node label>";
navigate {
page <page ref>{
filter(parameter, parameter);
}
}
//Possible child nodes of current node
connections {
node <node 1 name>(<Array ref or datasource for node 1>);
node <node 2 name>(<Array ref or datasource for node 2>);
....
node <node n name>(<Array ref or datasource for node n>);
}
}
//Node 2 definition
node <node 2 name> for <entity>{
label = "<node label>";
navigate {
page <page ref>{
filter(parameter, parameter);
}
}
//Possible child nodes of current node connections { node <node 1 name>(<Array ref or datasource for node 1>); node <node 2 name>(<Array ref or datasource for node 2>); .... node <node n name>(<Array ref or datasource for node n>); } } ...
//Node n definition node <node n name> for <entity>{ label = "<node label>";
page <page ref>{
filter(parameter, parameter);
}
}
//Possible child nodes of current node connections { node <node 1 name>(<Array ref or datasource for node 1>); node <node 2 name>(<Array ref or datasource for node 2>); .... node <node n name>(<Array ref or datasource for node n>); } } }
connections
section needs to be changed as described in the code example below.
...
connections {
node <node 1 name> using <function/entityset for node 1>;
node <node 2 name> using <function/entityset for node 2>;
....
node <node n name> using <function/entityset for node n>;
}
...
Limitations
The data source of the root node needs always to be the data source of the main record page to a context, but if there is no defined page to a context, this is created when selecting the context.
tree <TreeName> {
...
navicontexts {
<Context1_Name> {
label = "Context 1 Label";
}
<Context2_Name> {
label = "Context 2 Label";
}
...
<Contextn_Name> {
label = "Context n Label";
}
}
...
}
Declarative syntax - Contexts (in tree)
node <TreeNodeName> {
...
navigate {
when Context1 page <PageName1> {
filter(src_attribute_1, dest_src_attribute_1);
filter(src_attribute_2, dest_src_attribute_2);
...
filter(src_attribute_n, dest_src_attribute_n);
}
...
when Context_m page <PageName_m> {
filter(src_attribute_1, dest_src_attribute_1);
filter(src_attribute_2, dest_src_attribute_2);
...
filter(src_attribute_n, dest_src_attribute_n);
}//This is the default page. If you haven't defined a page to a context this will be executed on selecting that context
default page <PageName_def> {
filter(src_attribute_1, dest_src_attribute_1);
filter(src_attribute_2, dest_src_attribute_2);
...
filter(src_attribute_n, dest_src_attribute_n);
}
}
}
Declarative syntax - Contexts (in tree node) It is also possible to combine multiple contexts usingor
in the when
- statement.
when Context2 or Context4 page{
filter(src_attribute_1, dest_src_attribute_1);
filter(src_attribute_2, dest_src_attribute_2);
...
filter(src_attribute_n, dest_src_attribute_n)
}
Declarative syntax - Multiple contexts
Setting the tree node visibility
node <TreeNodeName> {
...
visible = [Context1 or Context2 or ... Context_n]
...
}
Declarative syntax - Tree node visibility The visible
property can be used to show/hide tree nodes, depending on the selected navigational context. Multiple navigational contexts can be set using the or
-operator. If the visible
property is not defined, then the tree node is by default set to visible.
2. Conditional navigations
Conditional navigations allow to use attributes of the record, root record or search context, to pick up the page dynamically.
node <TreeNodeName> {
...
navigate {
when [<Attribute1> = "<value>"] page <PageName1> {
filter(src_attribute_1, dest_src_attribute_1);
filter(src_attribute_2, dest_src_attribute_2);
...
filter(src_attribute_n, dest_src_attribute_n);
}
...
when [<Attribute_m> = "<value>"] page <PageName_m> {
filter(src_attribute_1, dest_src_attribute_1);
filter(src_attribute_2, dest_src_attribute_2);
...
filter(src_attribute_n, dest_src_attribute_n);
}
default page <PageName_def> {
filter(src_attribute_1, dest_src_attribute_1);
filter(src_attribute_2, dest_src_attribute_2);
...
filter(src_attribute_n, dest_src_attribute_n);
}
}
}
Declarative syntax - Conditional navigations
Using navigational contexts and conditional navigations together
Conditional navigations can also be used to combine multiple conditions withor
and and
in the when
-statement.
when [<Attribute1> = "<value>" and <Attribute2> != "<value>"] or Context1 page <PageName1> {
filter(src_attribute_1, dest_src_attribute_1);
filter(src_attribute_2, dest_src_attribute_2);
...
filter(src_attribute_n, dest_src_attribute_n);
}
Declarative syntax - Navigational contexts and conditional navigations.
3. Use of search contexts
Similar to a functions as parameters, and do filtering on pages loaded from tree nodes.Filtering tree nodes using search context
tree <TreeName> using <Entityset> {
label = "<Label>";
searchcontext <SearchContextName>{
defaults = <FunctionWichReturnsDefaultValues>;
}
selector <SelectorName>;
....
}
Declarative syntax - Search context filter (in tree).
tree <TreeName> using <EntitySet> {
...
node <node1Name> for <Entity> {
...
connections {
node <node2name> using <Function/Entityset> {
defaultfilter = [<Attribute1> = <SearchContextName>.<Field1> and ... <Attribute_n> = <SearchContextName>.<Field_n>];
}
}
}
}
Declarative syntax - Search context filter (in tree node).
rootnode RootNode {
label = "HierarchyId - ${HierarchyId} - ${Description}";
navigate {
page CustomerHierarchyPage {
filter(HierarchyId, HierarchyId);
filter(NumTest, NumTest);
}
}
connections {
node SubCustNode(CustomerHierarchyArray) {
defaultfilter = [HierarchyId = CustomerTreeFilter.HierarchyId];
}
}
}
Example code - Search context filter (in tree node) The property defaultfilter
can be used with any data source(function, entity set and array). Besides using search context fields in a defaultfilter
statement, they can also be used as parameters of functions.
rootnode RootNode {
label = "HierarchyId - ${HierarchyId} - ${Description}";
navigate {
page CustomerHierarchyPage {
filter(HierarchyId, HierarchyId);
filter(NumTest, NumTest);
}
}
connections {
node SubCustNodeusing GetCustomers(CustomerTreeFilter.HierarchyId)
}
}
Example code - Search context fields as parameters of a function
4. Copy/Move nodes in the tree control
The feature is provided to restructure the tree control at run time. This allows the user to copy and move the tree nodes.- Click the Edit button to enable the edit mode of the tree, see Figure 4.
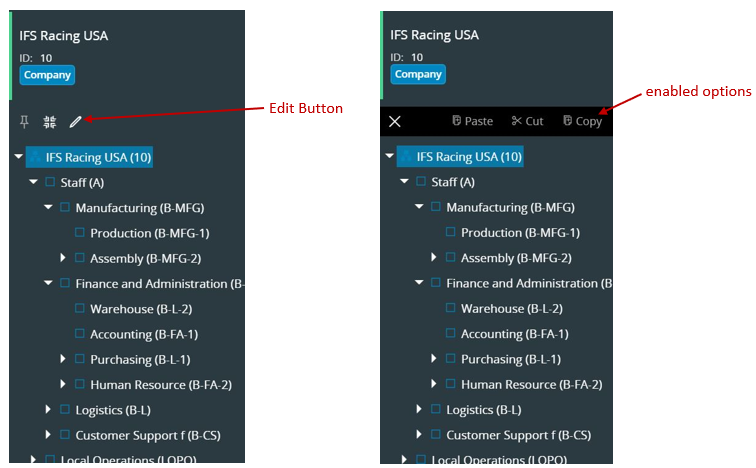
command <Command_Name> for <Entity> {
label = "<label>";
...
call <action_name>(source.param_1, source.param_2, ..., source.param_n, destination.param_1, destination.param_2, ..., destination.param_m);
...
}
Declarative syntax - Command definition Parameters of the action can be passed from both source node (node which is being copied or moved) and destination node (node where the copied or moved node goes to). In this case source and destination keywords can be used to access these nodes.
onmove {
when <Parent_Node_1> command <Command_1>;
when <Parent_Node_2> command <Command_2>;
...
when <Parent_Node_n> command <Command_n>;
}
oncopy {
when <Parent_Node_1> command <Command_1>;
when <Parent_Node_2> command <Command_2>;
...
when <Parent_Node_n> command <Command_n>;
}
Declarative syntax - Tree node
action ChangeParentCustomer Text {
ludependencies = CustHierarchyStruct;
parameter CustomerNo Text;
parameter NewParent Text;
}
Example code - Projection file
FUNCTION Change_Parent_Customer (
customerno_ IN VARCHAR2,
new_parent_ IN VARCHAR2 ) RETURN VARCHAR2
IS
BEGIN
UPDATE cust_hierarchy_struct_tab
SET customer_parent = new_parent_
WHERE customer_no = customer_no_;
RETURN 'SUCCESS';
END Change_Parent_Customer_;
Example code - Plsvc file
tree CustomerHierarchyTree using CustomerHierarchys {
label = "Customer Hierarchy";
searchcontext CustomerTreeFilter {
defaults = GetDefaultValuesForTreeFilter();
}
selector CustomerHierarchySelector;
command GoToTree;
rootnode RootNode {
label = "HierarchyId - ${HierarchyId} - ${Description}";
navigate {
page CustomerHierarchyPage {
filter(HierarchyId, HierarchyId);
}
}
connections {
node SubCustNode(CustomerHierarchyArray);
}
}
node SubCustNode for CustHierarchyStruct {
label = "Customer - ${CustomerNo} ";
orderby = CustomerNo asc;
navigate {
page CustHierarchyStructPage {
filter(CustomerNo, CustomerNo);
}
}
onmove {
when RootNode command ChangeParentCustomer;
when SubCustNode command ChangeParentCustomer;
}
oncopy {
when RootNode command CopyCustomer;
when SubCustNode command CopyCustomer;
}
connections {
node SubCustNode(ChildArray);
}
}
}
command ChangeParentCustomer for CustHierarchyStruct {
label = "Change Parent Customer";
execute {
call ChangeParentCustomer(source.CustomerNo, destination.CustomerNo);
}
}
Example code - Client file
5. Navigational parameters
When a page which comes from a tree control.
node OrderLineNode for CustomerOrderLine {
label = "Ord ${OrderNo} - Line ${LineNo}";
navigate {
page CustomerOrderLinePage {
filter(OrderNo, OrderNo);
filter(LineNo, LineNo);
}
}
}
Example code - Filter parameter
searchcontext
Page search context parameters can be passed using this syntax. This is not a mandatory navigational parameter.
navigate {
page CustomerHierarchyPage {
filter(HierarchyId, HierarchyId);
searchcontext(HierarchyId, Id);
searchcontext(Date, StartDate);
}
}
Example code - Searchcontext parameter
context
Global contexts of the page can be set using this syntax. This is also an optional parameter.
navigate {
page CustomerHierarchyPage {
filter(HierarchyId, HierarchyId);
context(CompanyId, Company);
context(SiteName, Site);
}
}
Example code - Context parameter
6. Using pages from other client files
A page with tree nodes can also be reused from other client files. This feature was introduced to reduce the length of the.client and.projection files of the tree control. The following syntax is used to refer files from other clients:
navigate {
page <client_name>.<page_name> {
filter(<attr1>, <attr_page1>);
filter(<attr2>, <attr_page2>);
...
}
}
Declarative syntax - Reference a client file
node OrderLineNode for CustomerOrderLine {
label = "Ord ${OrderNo} - Line ${LineNo}";
navigate {
page CustomerOrderInfo.CustomerOrderLinePage {
filter(OrderNo, OrderNo);
filter(LineNo, LineNo);
}
}
}
Example code - Reference a client file
7. Icons
Icons can be used in the tree structure to easily distinguish between different nodes. By default there are no icons for tree nodes available, but aniconset
can be used and have several icon expressions and emphasis values.
iconset {
icon "<icon_name1>" {
expression = [<expression1>];
}
icon "<icon_name2>" {
expression = [<expression2>];
}
...
icon "<icon_name3>";
emphasis <color_name1> = [<color_expression1>];
emphasis <color_name2> = [<color_expression2>];
...
}
Declarative syntax - Icon expressions and emphasis values Note! An expression
and emphasis
value is optional, only the icon name is mandatory. Only the icon values from the icon library can be used. The order of the expressions matters, therefore the first expression which is evaluated to be true takes effect. An icon definition without an expression always evaluates to be true. It is recommended to use such expressions after defining all the other expressions.
iconset {
icon "circle-solid" {
expression = [HierarchyId = "*"];
}
icon "business-structure" {
expression = [HierarchyId = "SL1"];
}
// icon value without an expression evaluates to be true
icon "business-structure-alt";
emphasis Complementary1 = [HierarchyId = "SL1"];
}
Example code - Icon expressions and emphasis values Tree searchcontext
values can also be used to evaluate the expressions and emphasis values of the icons.
iconset {
icon "circle-solid" {
expression = [CurrentStatus = CustomerTreeFilter.Status];
}
emphasis Complementary6 = [CurrentStatus = CustomerTreeFilter.Status];
}
Example code - Icon expressions and emphasis values (searchcontext)